プログラム言語 リスト・連想配列
目次
リスト(コレクション)
リストの定義
リストの型については「プログラム言語 ジェネリック」参照
リスト、コレクションの定義は言語によって違う
ここではイミュータブル(変更不可)な配列として扱う
ミュータブルな配列については
「プログラム言語 配列」参照
タプル(イミュータブル)な配列
リストの操作
初期化
$array = [
[
'id' => 5,
'name' => 'php',
],
[
'id' => 10,
'name' => 'python',
],
];
配列のインデックスは自動で振られる
[
0 => [
'id' => 5,
'name' => 'php',
],
1 => [
'id' => 10,
'name' => 'python',
],
]
collect関数を利用
$collection = collect($array);
Collectionインスタンスに変換される
object(Illuminate\Support\Collection) {
items [
[
0 => [
'id' => 5,
'name' => 'php',
],
1 => [
'id' => 10,
'name' => 'python',
],
],
]
}
これはEloquentを利用した場合の結果と同じ
Eloquentについては「プログラム言語 データベース操作 ORM」参照
List list = new ArrayList<>();
list.add(1);
list.add(1);
list.add(2);
list.add(3);
list.add(5);
List
要素の追加・変更・削除不可
ArrayListと別型
List
要素の追加・変更・削除可
ArrayListと同型
tuple = (1, 2, 3)
↓ も可
tuple = 1, 2, 3
配列、算術パッケージ
import numpy as np
ary = np.asarray([1, 2, 3])
print(ary) # [1 2 3]
整数
int = np.asarray([1.5, 2.5, 3.5], dtype=np.int32)
print(int) # [1 2 3]
浮動小数点数型
float = np.asarray([1.5, 2.5, 3.5], dtype=np.float)
print(float) # [1.5 2.5 3.5]
変換
float = int.astype(np.float32)
print(float) # [1. 2. 3.]
要素数
print(ary.shape) # (3,)
要素が0のリスト
ary = np.zeros(10, np.int32)
print(ary) # [0 0 0 0 0 0 0 0 0 0]
ary = np.ones(10, np.int32)
print(ary) # [1 1 1 1 1 1 1 1 1 1]
1未満の乱数
ary = np.random.rand()
print(ary) # 0.05840233316803445
1未満の乱数 & 3要素のリスト
ary = np.random.rand(3)
print(ary) # [0.29455163 0.62542719 0.85194529]
文字列→リスト変換 / 文字列←リスト変換
print(‘Java php python C#’.split())
#[‘Java’, ‘php’, ‘python’, ‘C#’]
print(‘Java, php, python, C#’.split())
#[‘Java,’, ‘php,’, ‘python,’, ‘C#’]
print(‘Java, php, python, C#’.split(‘,’))
#[‘Java’, ‘ php’, ‘ python’, ‘ C#’]
print(‘Java,php,python,C#’.split(‘,’))
#[‘Java’, ‘php’, ‘python’, ‘C#’]
リストのコピー
ary2 = ary1 #参照元をコピー
ary2[1] = ‘C#’ #参照元を変更
print(ary1) #[‘php’, ‘C#’, ‘Python’]
print(ary2) #[‘php’, ‘C#’, ‘Python’]
ary1 = [‘php’,’Java’,’Python’]
ary2 = ary1.copy() #値をコピー
ary2[1] = ‘C#’
print(ary1) # [‘php’, ‘Java’, ‘Python’]
print(ary2) # [‘php’, ‘C#’, ‘Python’]
ary1 = {‘php’:’CakePHP3′, ‘Java’:’SpringBoot’, ‘Python’:’Django’}
ary2 = ary1 #参照元をコピー
ary2[‘php’] = ‘Larabel’ #参照元を変更
print(ary1) # {‘php’: ‘Larabel’, ‘Java’: ‘SpringBoot’, ‘Python’: ‘Django’}
print(ary2) # {‘php’: ‘Larabel’, ‘Java’: ‘SpringBoot’, ‘Python’: ‘Django’}
ary1 = {‘php’:’CakePHP3′, ‘Java’:’SpringBoot’, ‘Python’:’Django’}
ary2 = ary1.copy() #値をコピー
ary2[‘php’] = ‘Larabel’
print(ary1) # {‘php’: ‘CakePHP3’, ‘Java’: ‘SpringBoot’, ‘Python’: ‘Django’}
print(ary2) # {‘php’: ‘Larabel’, ‘Java’: ‘SpringBoot’, ‘Python’: ‘Django’}
ary1 = [[‘php’, ‘Java’, ‘Python’],[‘CakePHP3’, ‘SpringBoot’ ,’Django’]]
ary2 = ary1 #参照元をコピー
ary2[1][0] = ‘Larabel’
print(ary1) # [[‘php’, ‘Java’, ‘Python’], [‘Larabel’, ‘SpringBoot’, ‘Django’]]
print(ary2) # [[‘php’, ‘Java’, ‘Python’], [‘Larabel’, ‘SpringBoot’, ‘Django’]]
ary1 = [[‘php’, ‘Java’, ‘Python’],[‘CakePHP3’, ‘SpringBoot’ ,’Django’]]
ary2 = ary1.copy() #配列の中の配列をコピー → 失敗
ary2[1][0] = ‘Larabel’
print(ary1) # [[‘php’, ‘Java’, ‘Python’], [‘Larabel’, ‘SpringBoot’, ‘Django’]]
print(ary2) # [[‘php’, ‘Java’, ‘Python’], [‘Larabel’, ‘SpringBoot’, ‘Django’]]
import copy
ary1 = [[‘php’, ‘Java’, ‘Python’],[‘CakePHP3’, ‘SpringBoot’ ,’Django’]]
ary2 = copy.deepcopy(ary1) #配列の中の配列をコピー → 成功
ary2[1][0] = ‘Larabel’
print(ary1) # [[‘php’, ‘Java’, ‘Python’], [‘CakePHP3’, ‘SpringBoot’, ‘Django’]]
print(ary2) # [[‘php’, ‘Java’, ‘Python’], [‘Larabel’, ‘SpringBoot’, ‘Django’]]
多次元
ary = [[1,2,3], [4,5,6]]
print(ary)
# [[1 2 3] [4 5 6]]
2つ目の配列の1つ目の要素を変更
ary[1][0] = 10
print(ary[1][0]) #10
配列、算術パッケージ
import numpy as np
多次元配列
ary = np.asarray([[1,2,3], [4,5,6]])
print(ary)
# [[1 2 3] [4 5 6]]
要素数
print(ary.shape)
# (2, 3)
1未満の乱数
ary = np.random.rand()
print(ary) # 0.05840233316803445
1未満の乱数 & 3要素の配列
ary = np.random.rand(3)
print(ary) # [0.29455163 0.62542719 0.85194529]
0以上1未満の乱数 & 2次元3要素の配列
ary = np.random.rand(2, 3)
print(ary) # [[0.40308448 0.73320688 0.52510381][0.983294 0.10888467 0.02262024]]
空リスト判定
$collection->isEmpty();
true
$collection->isNotEmpty();
false
$collection = collect([]);
$collection->isEmpty();
true
$collection->isNotEmpty();
false
boolean bool1 = CollectionUtils.isEmpty(list);
true
boolean bool2 = CollectionUtils.isNotEmpty(list);
false
要素の操作
追加・結合・削除
$array1 = [
[
'id' => 5,
'name' => 'php',
],
];
$array2 = [
[
'id' => 10,
'name' => 'python',
],
];
$collection = collect($array1)->merge(collect($array2));
object(Illuminate\Support\Collection) {
items [
[
0 => [
'id' => 5,
'name' => 'php',
],
1 => [
'id' => 10,
'name' => 'python',
],
],
]
}
要素が重複している場合はmerge先(この場合$array2の値で上書きされる)
List myList = new List<int> {10,20,30,40,50};
var myList = new List {10,20,30,40,50}; も可
追加
myList.Add(6);
int[] myInt = { 1, 2, 3 };
var list = new List<string>() { @”A”, @”B” };
list.AddRange(myInt.Select(n => n.ToString()));
list.AddRange(Enumerable.Range(start: 4, count: 10).Select(n => n.ToString()));
結合して追加
int[] ary1 = { 1, 2, 3 };
int[] ary2 = { 4, 5, 6 };
ary1 = ary1.Concat(ary2).ToArray();
削除
myList.Remove(10);
myList.Remove(myList[3]);
myList.RemoveAt(1);
初期化
myList.Clear();
int main(array<System::String ^> ^args)
{
ベクター(コレクション)
std::vector<int> myVector;
イテレーター:位置情報
std::vector<int>::iterator myBegin;
std::vector<int>::iterator myEnd;
myVector.push_back(10); // 末尾に追加
myVector.push_back(20); // 末尾に追加
myVector.push_back(30); // 末尾に追加
myVector.pop_back(); // 末尾を削除
Console::WriteLine(myVector[0]);
Console::WriteLine(myVector.at(0));
// → 10
// 先頭要素の取得(削除はしない)
int elm = myVector.front();
// elm:10
// 末尾要素の取得(削除はしない)
int elm = myVector.back();
// elm:30
myBegin = myVector.begin(); //最初の要素位置
myEnd = myVector.end(); //最後の要素位置
myFunc(myBegin, myEnd);
return 0;
}
void myFunc(std::vector<int>::iterator prmBegin, std::vector<int>::iterator prmEnd)
{
// 位置情報:先頭~末尾まで全件Loop
for (std::vector<int>::iterator tmp = prmBegin; tmp != prmEnd; tmp++)
{
Console::WriteLine(*tmp);
}
↓も同じ
// 位置情報:先頭~末尾まで全件Loop
for (; prmBegin != prmEnd; ++prmBegin)
{
// ポインタを用いて遠隔操作
Console::WriteLine(*prmBegin);
}
}
myList.Add(15)
myList.Remove(20)
myList.Remove(myList(2))
myList.RemoveAt(1)
myList.Sort()
myList.Clear()
IF | クラス | 順序 | 重複 | 追加 | 削除 | 参照 | |
---|---|---|---|---|---|---|---|
Collection | List | ArrayList | 格納順 | 〇 | add | remove | — |
SortedList | 整列 | 〇 | add | remove | — | ||
Set | HashSet | 順不同 | × | add | remove | — | |
TreeSet | 整列 | × | add | remove | — | ||
Queue | ArrayDeque | FIFO | 〇 | offerofferLast | poll | peek | |
Deque | ArrayDeque | LIFO | 〇 | offerFirst | poll | peek | |
Map | Map | HashMap | 順不同 | キー:×値:〇 | put | remove | get(キー) |
TreeMap | 整列 | キー:×値:〇 | put | remove | get(キー) |
ArrayList
List<String> list = new ArrayList<>();
list.add(“1”);
list.add(“2”);
list.add(“2”);
list.add(“3”);
for (String key : list){
System.out.println(key);
}
→1 2 2 3
追加
ArrayList array = new ArrayList<>();
ArrayList<Object> array = new ArrayList<Object>();と同じ
array.add(5);
⇒ array {5}
array.add(“5”);
⇒ array {5,”5″}
array.add(‘5’);
⇒ array {5,”5″,’5′}
指定の位置に追加
array.add(2,5);
⇒ array {5,”5″,5,’5′}
先頭から合致した要素を上書き
array.set(0, 50);
⇒ array {50,”5″,5,’5′}
先頭から合致した要素を削除
array.remove(“5”);
⇒ array {50,5,’5′}
条件に合致した要素を削除
List
list.removeIf(i -> i.equals(5));
list.toString();
[1, 2, 3, 4, 6, 7, 8, 9, 10]
for (int i=0; i<array.size(); i++){
String s = array.get(i);
}
for (Iterator i=array.iterator(); i.hasNext(); ){
String s = i.next().toString();
}
HashSet
HashSet<String> hashSet = new HashSet<>();
hashSet.addAll(list);
for (String key : hashSet){
System.out.println(key);
}
→2 1 3
TreeSet
TreeSet<String> treeSet = new TreeSet<>();
treeSet .addAll(list);
for (String key : treeSet){
System.out.println(key);
}
→1 2 3
Queue
Queue<String> queue = new ArrayDeque<>();
queue.addAll(list);
System.out.println(queue.poll());
System.out.println(queue.poll());
queue.offer(“2”);
System.out.println(queue.poll());
System.out.println(queue.poll());
System.out.println(queue.poll());
→1 2 2 3 2
Deque
Deque<String> stack = new ArrayDeque<>();
stack.offerFirst(“1”);
stack.offerFirst(“2”);
stack.offerFirst(“2”);
stack.offerFirst(“3”);
System.out.println(stack.poll());
System.out.println(stack.poll());
stack.offerFirst(“2”);
System.out.println(stack.poll());
System.out.println(stack.poll());
System.out.println(stack.poll());
→3 2 2 2 1
ary = [‘php’, ‘python’, ‘Java’]
# 末尾に追加
ary += [‘C#’]
print(ary)
→[‘php’, ‘python’, ‘Java’, ‘C#’]
# 追加
ary = []
ary.append(‘php’)
ary.append(‘python’)
ary.append(‘Java’)
ary.append(‘C#’)
print(ary)
→[‘php’, ‘python’, ‘Java’, ‘C#’]
# インデックスを指定して追加
ary = []
ary.insert(0, ‘php’) #先頭に追加
ary.insert(1, ‘python’) #2番目に追加
ary.insert(5, ‘Java’) #末尾に追加
print(ary)
→[‘php’, ‘python’, ‘Java’]
削除
ary.remove(‘php’)
del ary[1] #インデックスを指定
del ary[-1] #末尾の要素を指定
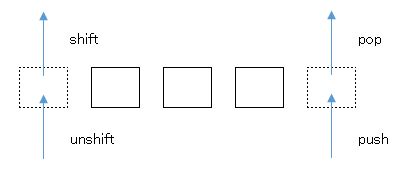
push
配列の最後に要素を追加する
my @x;
push(@x, 1);
my @y = (2,3);
push(@x, @y);
push(@x, 4, 5); #連続でいくつでも追加可
⇒ @x : 1 2 3 4 5
pop
配列の最後の要素を取り除く
my @before = (1,2,3,4,5);
pop(@before);
⇒ @before : 1 2 3 4
my $after = pop(@before);
⇒ $after : 4
⇒ @before : 1 2 3
shift
配列の最初の要素を取り除く
my @before = (1,2,3,4,5);
shift(@before);
⇒ @before : 2 3 4 5
my $after = shift(@before);
⇒ $after : 2
⇒ @before : 3 4 5
unshift
先頭へ要素追加
my @x;
my @y = (1,2,3);
unshift(@x, @y);
unshift(@x, 0, -1);
⇒ @x : -1 0 1 2 3
splice
配列から指定の要素を、指定の個数分取り除く
splice(配列 , 開始Index , 個数);
my @x = (1,2,3,4,5);
splice(@x,0,2);
⇒ @x : 3 4 5
reverse
配列の要素を逆順にする
my @before = (1,2,3,4,5);
my @after = reverse(@before);
⇒ @before : 1 2 3 4 5
⇒ @after : 5 4 3 2 1
sort
配列の要素をソートする(数値限定)
my @before = (2,1,4,3,5);
昇順
my @after = sort{$a <=> $b}(@before);
⇒ @before : 2,1,4,3,5
⇒ @after : 1 2 3 4 5
降順
my @after = sort{$b <=> $a}(@before);
⇒ @before : 2,1,4,3,5
⇒ @after : 5 4 3 2 1
置換
list.replaceAll(i -> i * 10);
list.toString():[10, 20, 30, 50, 70]
比較
Comparable | Comparator | |
---|---|---|
機能 | 自身と他者との大小比較 | 2オブジェクトの大小比較 |
Package | java.lang.Comparable | java.util.Comparator |
メソッド | compareTo |
・compare ・equals ・reversed |
クラス |
・Integer ・String等 |
・TreeSet ・TreeMap等 |
package java.lang;
public interface Comparable<T> {
public int compareTo(T o);
}
例
public final class Integer extends Number implements Comparable<Integer> {
public int compareTo(Integer anotherInteger) {
return compare(this.value, anotherInteger.value);
}
public static int compare(int x, int y) {
return (x < y) ? -1 : ((x == y) ? 0 : 1);
}
}
package java.util;
public interface Comparator<T> {
int compare(T o1, T o2);
boolean equals(Object obj);
default Comparator<T> reversed() {
return Collections.reverseOrder(this);
}
}
検索
$array = [
[
'id' => 5,
'name' => 'php',
],
[
'id' => 10,
'name' => 'python',
],
];
$collection = collect($array);
$collection = $collection->filter(function($item){
return $item['name'] === 'php';
});
object(Illuminate\Support\Collection) {
items [
[
0 => [
'id' => 5,
'name' => 'php',
],
],
]
}
int found = Collections.binarySearch(list, “Java”);
0
int found = Collections.binarySearch(list, “Java”);
-5
計算
import numpy as np
ary = np.asarray([1, 2, 3])
# 合計
print(np.sum(ary))
# 平均
print(np.mean(ary))
# 最大値
print(np.max(ary))
# 最小値
print(np.min(ary))
# 標準偏差
print(np.std(ary))
並べ替え
自然順序:数字、アルファベット順等
$array = [
[
'id' => 5,
'name' => 'php',
],
[
'id' => 10,
'name' => 'python',
],
];
$collection = collect($array)->sortBy('id')->values();
$collection = collect($array)->sortByDesc('id')->values();
value()メソッド
キーが元のまま並べ替えされるのでキーを振り直す
string[] ary = new string[] { “b”, “aaaaa”, “cc” };
Array.Sort(ary, (x, y) => x.Length.CompareTo(y.Length));
※x, y には長さを比較する為の各要素が入り、2つの要素の長さ比較を行う
DateTime[] ary = new DateTime[]
{
new DateTime(year: 2010, month: 1, day: 1),
new DateTime(year: 2001, month: 1, day: 1),
new DateTime(year: 2005, month: 1, day: 1),
new DateTime(year: 2003, month: 1, day: 1)
};
Array.Sort(ary, (x, y) => Math.Sign(x.Ticks – y.Ticks));
var newary1 = ary.OrderBy(n => n);
list.add(“20”);
list.add(“30”);
list.add(“10”);
list:20 30 10
Collections.sort(list);
list:10 20 30
Collections.reverse(list);
list:30 20 10
並べ替え(定義)
private String _name;
private Integer _id;
public Employee(String name, Integer id){
this._name = name;
this._id = id;
}
public Integer getId(){
return this._id;
}
public String getName(){
return this._name;
}
}
※Integer.class
public int compareTo(Integer anotherInteger) {
return compare(this.value, anotherInteger.value);
}
public static int compare(int x, int y) {
return (x < y) ? -1 : ((x == y) ? 0 : 1);
}
import java.util.Comparator;
public class MyRule implements Comparator<Employee>{
public int compare(Employee obj1, Employee obj2){
return obj1.getId().compareTo(obj2.getId());
}
}
※java.util.Collections.class
public class Collections {
public static void sort(List list1) {
list1.sort(null);
}
public static void sort(List list1, Comparator comparator) {
list1.sort(comparator);
}
}
public class StudyMain {
public static void main(String[] args) {
Employee e1 = new Employee(“JavaScript”, 20);
Employee e2 = new Employee(“php”, 25);
Employee e3 = new Employee(“Java”, 10);
List<Employee> list = new ArrayList<>();
list.add(e1);
list.add(e2);
list.add(e3);
System.out.println(“ArrayListの格納順”);
print(list);
→20 JavaScript 25 php 10 Java
System.out.println(“MyRole.ID順”);
Collections.sort(list, new MyRule());
print(list);
→10 Java 20 JavaScript 25 php
}
private static void print(List<Employee> list){
for (Employee obj : list){
System.out.println(obj.getId() + ” ” + obj.getName());
}
}
}
抽出
$array = [
[
'id' => 5,
'name' => 'php',
],
[
'id' => 10,
'name' => 'python',
],
];
$collection = collect($array)->pluck('id');
[
0 => 5,
1 => 10,
];
キーは自動で振られる
キーを要素で指定
$collection = collect($array)->pluck('name', 'id');
[
5 => 'php',
10 => 'python'
,
];
ネストされた要素を抽出
$array = [
[
'id' => 5,
'language' => [
'name' => 'php',
'year' => 5,
],
],
[
'id' => 10,
'language' => [
'name' => 'python',
'year' => 3,
],
],
];
$collection = collect($array)->pluck('language.name', 'id');
[
5 => 'php',
10 => 'python'
,
];
連想配列(ハッシュ、ディクショナリ、マップ)
基本
dic.Add(1, @”西宮”);
dic.Add(2, @”神戸”);
dic.Add(3, @”芦屋”);
Dictionary<string, string> myDic = new Dictionary<string, string>
{
{“1Top”,”大久保”},
{“左Wing”,”香川”},
{“右Wing”,”岡崎”},
{“Top下”,”本田”}
};
foreach (var element in myDic){
string pos = element.Key;
string name = element.Value;
};
foreach (string myKey in myDic.Keys)
{
string pos = myKey;
string name = myDic[pos];
};
string ret;
myDic.TryGetValue(key: 3, value: out ret);
key:3が無ければnullが返る
ポインタ(リファレンス/デリファレンス)を使用した配列の利用については「プログラム言語 ポインタ / 連想配列(ハッシュ)のポインタ」参照
自動整列リスト
SortedList<int, string> mySorted = new SortedList<int, string>
{
{11,”大久保”},
{10,”香川”},
{9,”岡崎”},
{4,”本田”}
};
Map<String, String> hashMap = new HashMap<String, String>();
hashMap.put(“B”, “Java”);
hashMap.put(“C”, “php”);
hashMap.put(“A”, “JavaScript”);
for (Map.Entry<String, String> entry : hashMap.entrySet()){
System.out.println(entry);
}
→B=Java C=php A=JavaScript
TreeMap
Map<String, String> treeMap = new TreeMap<String, String>();
treeMap.put(“B”, “Java”);
treeMap.put(“C”, “php”);
treeMap.put(“A”, “JavaScript”);
for (Map.Entry<String, String> entry : treeMap.entrySet()){
System.out.println(entry);
}
→A=JavaScript B=Java C=php
マップの初期化(匿名関数)
※lassファイルが2つできてしまう為非推奨
public static final HashMap<String, String> pg = new HashMap<String, String>(){
{
put(“01”, “Java”);
put(“02”, “C#”);
put(“03”, “php”);
put(“04”, “JavaScript”);
}
};
マップの初期化(イニシャライザ)
private static Map<String, Double> exp;
static {
exp = new HashMap<>();
exp.put(“VB6”, 2D);
exp.put(“VB.Net”, 4D);
exp.put(“Java”, 3D);
exp.put(“C#”, 2D);
exp.put(“PHP”, 1D);
}
要素の追加
exp.put(“Python”, 0.5);
exp.toString();
{C#=2.0, Java=3.0, VB.Net=4.0, PHP=1.0, VB6=2.0, Python=0.5}
要素の削除
exp.remove(“VB6”);
exp.remove(“VB.Net”);
exp.toString();
{C#=2.0, Java=3.0, PHP=1.0, Python=0.5}
要素の取得
exp.get(“Java”));
3.0
要素の上書き
exp.put(“Java”, 4D);
キーが重複している場合
exp.get(“Java”);
4.0
要素数
exp.size());
4
キーの存在チェック
exp.containsKey(“Java”);
true
exp.containsKey(“Ruby”);
false
値の存在チェック
exp.containsValue(3.0);
true
exp.containsValue(10.0);
false
キーのLoop
for (String lungage : exp.keySet()) {
lungage;
C# Java PHP Python
}
値のLoop
for (Double year : exp.values()) {
year;
2.0 4.0 1.0 0.5
}
要素のLoop
for (Entry
map.getKey();
C# Java PHP Python
map.getValue();
2.0 4.0 1.0 0.5
}
指定した値が無い場合のデフォルト値を指定して取得
List<String> language = Arrays.asList(“Java”, “C#”, “PHP”, “C#”, “Python”, “Java”);
Map<String, Integer> counter = new HashMap<>();
language.forEach(s -> {
counterにキーが無い(==null)場合は0、あればキーに対応する値を取得
Integer i = counter.getOrDefault(s, 0);
従来の書き方
Integer i = counter.get(s) == null ? 0 : counter.get(s);
counter.put(s, i+1);
});
counter.forEach((key, value) -> {
System.out.println(key + “:” + value);
});
C#:2 Java:2 PHP:1 Python:1
{“key1”, “value1”}, _
{“key3”, “value3”}, _
{“key2”, “value2”}, _
{“key4”, “value4”}
}
For Each element In myDic
Dim key = element.Key
Dim value As String = element.Value
Next element
For Each element As String In myDic.Keys
Dim key As String = element
Dim value As String = myDic(key)
Next element
自動整列リスト
Dim mySorted As New SortedList(Of String, String)() From { _
{“key1”, “value1”}, _
{“key3”, “value3”}, _
{“key2”, “value2”}, _
{“key4”, “value4”}
}
Dim dic As New Scripting.Dictionary
dic.Add “key1”, “item1”
dic.Add “key2”, “item2”
dic.Add “key3”, “item3”
Dim key as Variant
For Each key In dic
debug.print dic.item(key)
→item1 item2 item3
Next key
キー全削除
dic.RemoveAll
※変数[キー] = 値;
var TestArray = new Object();
TestArray[“key1”] = value1;
TestArray[“key2”] = value2;
TestArray[“key3”] = function(){ alert(“value3です。”); };
alert(TestArray[“key1”]);
var strfunc = “key2”
alert(TestArray[strfunc]);
for (var i = 1; i <= 3; ++i) { alert["key" + i]; } ②var変数 = {キー:値 , キー:値, ・・・} var TestArray = { key1:value1, key2:value2, key3:function(){ alert("value3です。");} }
’php’ => ‘CakePHP’,
’java’ => ‘SpringBoot’,
’JavaScript’ => ‘node.js’,
];
print $list[‘php’];
→’CakePHP’
追加
$list += [
’php’ => ‘Laravel’,
];
dic = {
‘php’:’CakePHP3′,
‘python’:’Django’,
‘Java’:’Spring-boot’,
}
# 追加
dic[‘C#’] = ‘.NetCore’,
# 削除
del dic[‘C#’]
# 指定の値
print(dic.get(‘php’)) # CakePHP3
print(dic.get(‘python’)) # None
# キーが無い場合の値も指定
print(dic.get(‘python’, ‘?’)) # ?
# キー
print(dic.keys())
# dict_keys([‘php’, ‘python’, ‘Java’])
# 値
print(dic.values())
# dict_values([‘CakePHP3’, ‘Django’, ‘Spring-boot’])
# キーと値
print(dic.items())
# dict_items([(‘php’, ‘CakePHP3’), (‘python’, ‘Django’), (‘Java’, ‘Spring-boot’)])
for key in dic.keys():
print(key)
# php
# python
# Java
for value in dic.values():
print(value)
# CakePHP3
# Django
# Spring-boot
for item in dic.items():
print(item)
# (‘php’, ‘CakePHP3’)
# (‘python’, ‘Django’)
# (‘Java’, ‘Spring-boot’)
for key, value in dic.items():
print(key, value)
# php CakePHP3
# python Django
# Java Spring-boot
# 存在チェック
print(‘php’ in dic.keys()) # True
print(‘CakePHP3’ in dic.values()) # True
print(‘CakePHP3’ in dic.items()) # False
print((‘php’, ‘CakePHP3’) in dic.items()) # True
# Keyが存在しない場合のみ追加
dic.setdefault(‘php’, ‘Laravel’) # phpキーが存在するので追加されない
dic.setdefault(‘C#’, ‘.Net’) # C#キーが存在しないので追加される
print(dic)
# {‘php’: ‘CakePHP3’, ‘python’: ‘Django’, ‘Java’: ‘Spring-boot’, ‘C#’: ‘.Net’}
$profile{“name”} = “yone”
$profile{“age”} = 35
print “$profile{‘name’}\\n”;
⇒yone
ハッシュへの一括代入
my %profile = (
’name’ => ‘yone’,
’age’ => 35
);
又は
my %profile = (“name”,”yone”,”age”,35)
keys
※連想配列からキーを取得
my $key = keys(%profile);
for my $key ( keys(%profile) ){
$keyにはkeyだけが入る
}
values
※連想配列から値を取得
my $value = values(%profile);
for my $value ( values(%ary) ){
$valueにはvalueだけが入る
}
delete
※連想配列から指定のキーを削除
delete($profile{“age”});
追加
$profile{新key} = ~;
exists
if ( exists($profile{キー}) ) {
~
}
ハッシュxを全件Loop。キー⇒name、値⇒valueへ格納。
while ( (my $name, my $value) = each(%x)){
print “$name = $value”;
}
※name,value の変数名は変更可能
操作
キーと値変換
’php’,
’python’,
’JavaScript’,
];
$before → [
0 => ‘php’,
1 => ‘python’,
2 => ‘JavaScript’,
];
$after = array_flip($before);
$after → [
’php’ => 0,
’python’ => 1,
’JavaScript’ => 2,
]
並べ替え
自然順序:数字、アルファベット順等
’name’ => ‘abcde’,
’age’ => 12,
’email’ => ‘aaa@test.com’,
’sex’ => ‘male’,
];
値の昇順
asort($array);
$array
[
’email’ => ‘aaa@test.com’,
’name’ => ‘abcde’,
’sex’ => ‘male’,
’age’ => 12,
]
値の降順
arsort($array);
$array
[
’age’ => 12,
’sex’ => ‘male’,
’name’ => ‘abcde’,
’email’ => ‘aaa@test.com’,
]
キーの昇順
ksort($array);
キーの降順
krsort($array);
他
配列・リスト変換
5 => 555,
10 => 222,
]);
$array = $collection->toArray();
string[] array = oldList.ToArray();
array[0]:”A” / array[1]:”B” / array[2]:”C”
int[] ary = Enumerable.Range(start: 1, count: 26).ToArray();
int[] ary = Enumerable.Repeat(element: -1, count: 100).ToArray();
List<string> newList = array.ToList();
newList.AddRange(array); も可
List<String> list = Arrays.asList(ary);
→Java php JavaScript
list.set(2, “C#”);
→Java php C#
list.remove(2); →実行時エラー(変換時はサイズ固定)
’key1′ => ‘java’,
’key2′ => ‘php’,
’key3′ => ‘C#’,
];
$ret = array_values($ary);
$ret → [‘java’, ‘php’, ‘C#’];
# タプル → リスト
after = list(before)
after[0] = ‘C#’ # OK
print(after) # [‘C#’, ‘Java’, ‘Python’]
# リスト → タプル
before = [‘php’, ‘Java’, ‘Python’]
after = tuple(before)
after[0] = ‘C#’ # エラー
Iterator/IEnumerableインターフェイス
↓ for (int i=0; i<10; i++) { ~ } の3つ目の処理を省略した形
for (Iterator<String> iterator = list.iterator(); iterator.hasNext();) {
String element = iterator.next();
Iteratorは要素を変更できる
if (element == “C#”) iterator.remove();
}
System.out.println(list.toString());
[Java, PHP, Python]
IEnumerableインターフェイスはGetEnumerator()メソッドを実装しており、
これを実行するとIEnumrableインターフェイスを返す。
配列、リストは最初からのこのインターフェイスを継承している。
int[] myArray = new int[] { 1,3,5,7 };
//配列myArrayはIEnumerableを継承しているので、GetEnumerator()を実行できる。
//IEnumerableインターフェイスを返すのでmyEに格納できる
IEnumerator myE = myArray.GetEnumerator();
while(myE.MoveNext())
{
int val = (int)myE.Current;
Console.WriteLine(val);
⇒ 1 3 5 7
}
// IEnumerableを継承いれば↓ の様に書ける
foreach(int n in myArray)
{
Console.WriteLine(n);
⇒ 1 3 5 7
}
// リストも同様にIEnumerableを継承しているのでforeach()構文の使用が可能
ArrayList myList = new ArrayList() { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
foreach(int i in myList)
{
Console.WriteLine(i);
⇒ 1 2 3 4 5 6 7 8 9 10
}